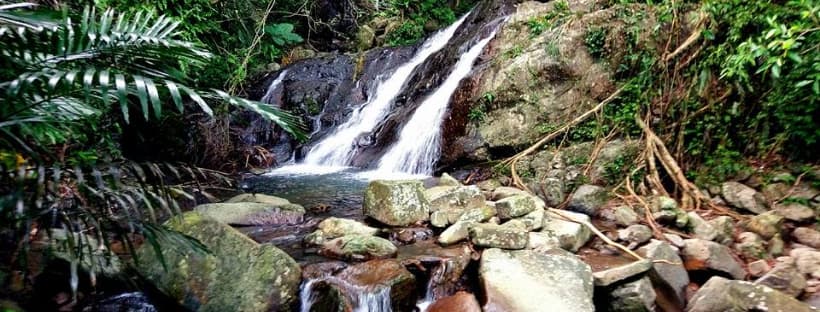
貼標籤。
1 2 3 4
| @Deprecated: 不建議使用的方法 @Override: 重寫父類 @SuppressWarnings({"deprecation", "unused","path"}) path: 忽略不存在路徑警告
|
#@FunctionallInterface
配合lambda的函數接口定義方式,一種特殊的interface。
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24
| // 定義Interface @FunctionalInterface interface TestAnnotation { String myApply(); }
// interface 實現 class Hi { void say(TestAnnotation annotation) { System.out.println(annotation.myApply()); } }
// 使用 public class Lambda { public static void main(String[] args) {
Hi hi = new Hi(); hi.say(() -> "Hello World"); } }
> Hello World
|
5個元注解
@Retention、@Documented、@Target、@Inherited、@Repeatable
- @Retention - 註解保留時間
- @Retention(RetentionPolicy.RUNTIME) - 會加載到JVM,運行時可用
- RetentionPolicy.CLASS - 不加載JVM,編譯時可用
- RetentionPolicy.SOURC - 不編譯,只存在程式碼
- @Documented - 在Javadoc會出現
- @Target - 指名註解地方
- ElementType.ANNOTATION_TYPE 給注解進行注解
- ElementType.CONSTRUCTOR
- ElementType.FIELD 屬性
- ElementType.LOCAL_VARIABLE 局部變數
- ElementType.METHOD
- ElementType.PACKAGE
- ElementType.PARAMETER 方法內參數
- ElementType.TYPE 類、接口、枚舉
- @Inherited - 子類繼承父類標籤
1 2 3 4 5 6 7 8
| @Inherited @Retention(RetentionPolicy.RUNTIME) @interface Test {}
@Test public class A {}
public class B extends A {}
|
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15
| @interface Persons { Person[] value(); }
@Repeatable(Persons.class) @interface Person{ String role default ""; }
@Person(role="artist") @Person(role="coder") @Person(role="PM") public class SuperMan{
}
|
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50
| // 類似介面的宣告方式 @interface TestAnnotation { int id(); String msg(); /* 或先宣告 public int id() default -1; public String msg() default "Hi"; */ }
// 使用 @TestAnnotation(id=3,msg="hello annotation") /* @TestAnnotation() */ public class Test { }
---
import java.lang.annotation.*; import java.util.Arrays;
@Target({ElementType.METHOD, ElementType.TYPE}) @Retention(RetentionPolicy.RUNTIME) @Documented @interface MyAnnotation { String[] value(); String comment() default "hello"; }
@MyAnnotation("John") class MyTest { public static void test(){ //透過反射取得某個類上的某個注解 MyAnnotation config = MyTest.class.getAnnotation(MyAnnotation.class); System.out.println("value: " + Arrays.toString(config.value()) + ", comment: " + config.comment()); } }
public class Annotation{ public static void main(String[] args) { MyTest.test(); //輸出 value: John, comment: hello } }
> value: [John], comment: hello
|
Annotations, OSI model and more
- 會話層(比如 Spring Bean、EJB 等): 代替 XML 配置文件
- 傳輸+ 網路層(TCP/IP): 路由映射、請求參數綁定等: Spring MVC 的 @RequestMapping
- 資料庫持久層與實體對象: JPA 的 @Entity、@Table
- 驗證參數的規則配置: Bean Validation 的 @NotNull、@Size 等
- 依賴注入的標示: Spring 的 @Autowired、@Qualifier 等
- AOP 方法的標记,定義增強邏輯: Spring 的 @Before、@After 等
- 測試用例的標示: JUnit 的 @Test、@BeforeClass 等