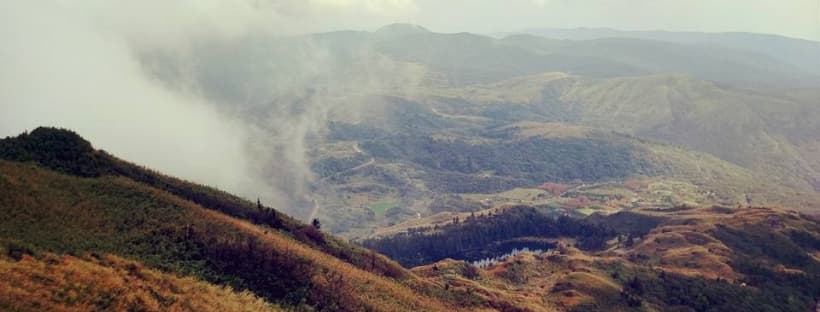
主流Java框架Spring。
系列文章
O
將之前學到的技術整理成系列文章,Java Spring Framework, Hibernate與Kubernetes。
KRs
- 領域探索 100%
簡化 Spring的框架,基於 Spring Framework (下面會提到),開發人員提供了一個快速、簡單、高效、可擴展和易於部署的開發框架,有助於加快開發進程,降低開發成本。
通過 Spring Boot Starter 模組(Maven 或 Gradle)實現自動配置,MySQL、MongoDB,消息隊列等支持,自動配置類中通常會註冊一些 Spring Bean,這些 Bean 會根據 Spring Boot 的自動配置規則進行設置。
application.properties實現對不同環境的配置支持。

Source Code
去除繁瑣配置,Spring MVC > Spring Boot取代
- 配置Maven依賴 >
Spring Boot保留
- 配置web.xml加載spring mvc
- 資料庫配置
- 讀取文件的配置
- Log
- Tomcat >
Spring Boot內建
Spring Annotations
- @SpringBootApplication:標記主配置類,同時啟用自動配置和組件掃描。
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32
| 2. @RestController:標記一個類為 REST 風格的控制器。 3. @GetMapping/@PostMapping/@PutMapping/@DeleteMapping:定義@RestController中 HTTP 的 GET/POST/PUT/DELETE 方法對應的路徑和處理器方法。 4. @Component:通用的組件注解,標記一個類為 Spring 組件。 5. @EnableCaching:啟用緩存管理。 6. @EnableAsync:啟用異步方法調用。 7. @Entity:對應到table, 後接@Table(name = "...") 8. @Autowired:自動注入 Spring Bean。 9. @Service:定義比CRUD更複雜操作 10. @SpringBootApplication: 啟動程式 11. @Data:Lombok 簡化 getter, setter, toString 12. @Repository封裝後端database crud操作 13. @Id @GeneratedValue(strategy = GenerationType.IDENTITY) private Long id; 14. @Transactional 實現事務管理,方法執行過程中發生異常,則事務會自動回滾,保證數據庫的一致性。
常用的事務管理器包括:
DataSourceTransactionManager:用於支持關聯到單個數據源的事務管理。 JtaTransactionManager:用於支持全局事務管理。 JpaTransactionManager:用於支持 JPA 操作的事務管理。
@Service public class UserService { @Autowired private UserRepository userRepository;
@Transactional public void addUser(User user) { userRepository.save(user); } }
|
配對應用程式模型物件和數據庫表格的資料的框架
@Entity
- Hibernate在POJO(Java 物件)上@就可以直接映射,MyBatis較複雜
- Hibernate 封裝SQL開發較快
- MyBatis 一對一或一對多的關聯設定較簡單
- MyBatis 可以對SQL優化查詢
專案建置
- Spring MVC(Model. View. Controller)
建構專案初始架構與相依套件
- 常用
- Spring Web(Spring MVC)
- Spring Data JPA(Hibernate)
- H2(In memory database)
- MySQL Driver
- Lombok
建置套件
Maven:XML
Gradle:Json
pom.xml添加新依賴後需要再
1
| ~/apache-maven-3.9.0/bin/mvn clean install
|
使用者由網頁切入(VCM)
- View: Postman( Get, Post, Put, Delete)
in Thymeleaf/JSP or command
- Controller: API Layer
以前是Struts負責,溝通前端JSP(View)與後端Servlets(Model)
- Model: Service(Business Logic)
資料數中的一個表
- Data Access Object, DAO: Presistence Logic
Hibernate封裝SQL,封裝資料庫進行持久層的操作
- DB
執行SQL語句
工程師由Model切入(MCV)
如果要添加MySQL到專案
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71
| 1. pom.xml: 添加依賴套件
<dependency> <groupId>org.springframework.boot</groupId> <artifactId>spring-boot-starter-jdbc</artifactId> </dependency>
<dependency> <groupId>mysql</groupId> <artifactId>mysql-connector-java</artifactId> <scope>runtime</scope> </dependency>
2. application.yml: 設置參數
server: port: 9100 # 伺服器的port號
spring: datasource: url: jdbc:mysql://localhost:3306/ems?useSSL=false # mysql databases 連接位址 username: root # database 用戶名 password: 123456 # database 密碼
jpa: properties.hibernate.dialect: org.hibernate.dialect.MySQL8Dialect hibernate.ddl-auto: update generate-ddl: true show-sql: true
3. `Model`: @Entity 對應到 MySQL 數據庫中的一個表
@Entity @Table(name="employees") public class Employee {
4. Respository: 繼承 JpaRepository 的interface
使用 Spring Data JPA 透過底層默認框架Hibernate來映射SQL語句,操作資料庫
5. Service: 定義比CRUD更複雜操作,像listUser...
@Service public class EmployeeServiceImpl implements EmployeeService {
private EmployeeRepository employeeRepository; … 6. Exception: Service會用到的錯誤控制
ResourceNotFoundException <class>
7. `Controller`: API接口定義
@RestController @RequestMapping("/api/employees") public class EmployeeController {
private EmployeeService employeeService; @RequestMapping("/api/employees") public class EmployeeController {
8. `View`: 透過內建Tomcat網頁呈現
9. 啟動程式
@SpringBootApplication public class DemoApplication {
public static void main(String[] args) {
|
- Lombok
簡化 getter, setter, toString等
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32
| 選擇Lombok或手動添加
<dependency> <groupId>org.projectlombok</groupId> <artifactId>lombok</artifactId> <version>1.18.0</version> </dependency>
使用
@Data public class Student {
會引入
import lombok.*;
或使用
@Getter @Setter @AllArgsConstructor @EqualsAndHashCode public class Student {
會引入
import lombok.AllArgsConstructor; import lombok.EqualsAndHashCode; import lombok.Getter; import lombok.Setter;
|
Spring Framework
整合簡化JSP、Servlet等Web Framework,解決類別間相依性
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15
| 選擇web與Template Engines或手動添加
<dependency> <groupId>org.springframework.boot</groupId> <artifactId>spring-boot-starter-thymeleaf</artifactId> </dependency> <dependency> <groupId>org.springframework.boot</groupId> <artifactId>spring-boot-starter-web</artifactId> </dependency>
引入後resource資料夾下多個Templates放模板
http://localhost:63342/spring5webapp/bookList.html
|

Spring的設計模式(IoC-DI, AOP)
設計原則,取代new物件的依賴
讓物件的產生與配置委託給IOC容器(這裡說的也就是Spring IOC Container),讓程式到了需要時,讓容器來幫我們注入
Object obj = new Object();
改成
Object obj = ApplicationContext.getBean(Object.class);
實現控制反轉,讓一個物件和 class接收它所依賴(變數)的其他物件,用Annotation分離接收方和依賴,從而提供鬆耦合以及代碼重用性。
A對B依賴,B改動時,A就要跟著改
1 2 3 4
| public class A{ B b = new B(); ... }
|
外部實例再傳入,方便維護
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15
| public class A { B b; public A(B _b) { this.b = _b; } public void a(B _b) { this.b = _b; ... } }
|
AOP(Aspect Oriented Programming with Spring): 一種概念與一系列標準,解決跨越多個模塊的重複性問題,例如日誌輸出、性能統計、安全控制等。
1 2 3 4 5 6 7 8 9
| 通過 @EnableAspectJAutoProxy 注解來啟用 AOP。
@SpringBootApplication @EnableAspectJAutoProxy public class DemoApplication { public static void main(String[] args) { SpringApplication.run(DemoApplication.class, args); } }
|
Proxy Design(代理模式): 預測使用者行為,自動裝配
- 靜態代理(Static Proxy): 程式還沒運行前就確立了整個物件的包裝與邏輯
- 動態代理(Dynamic Proxy): 代理物件交由程式運行時生成
Spring Boot 中最常用的微服務框架之一,它基於 Spring Boot 構建,提供了一系列開箱即用的微服務組件,包括服務發現、負載均衡、配置中心、網關等,使得開發者可以更輕鬆地實現微服務架構,Eureka、Ribbon、Zuul、Config Server 等。
時程: 2023/2/1- 2023/3/1
Refs
- Annotation取代實作interface
- POJO
- SSH(Spring + Struts + Hibernate)